I Wanna Dive Into WebGL:
Let's Do This!
So, a little reminder - in order to create WebGL experiences, you'll need to know how to write Javascript code. While the learning curve isn't as hard as it seems, it's still something to consider.
BTW, there's some exceptions to this - we'll go over them soon.
Another important thing to understand is that all files that your WebGL experience consists of need to be hosted online.
This could be a Web Server you create, or a Cloud Service you use - as long as you have an HTTPS address of your files that you can link directly into WIX's HTML iframe (remember that Website Address option from before?).
For the sake of this lecture we're going to focus on the Cloud option, since it's much more simple and straight forward. You can use Google Drive or Dropbox, or something more professional like Amazon AWS.
But first, let's talk about index.html...
WebGL & Index.html
Whether you're creating your WebGL experience using Babylon JS or Unity (which we'll explain later-on what they are exactly), you're going to have (roughly) 2 types of files:
-
An index.html file.
-
The assets used in your WebGL experience (3D models, textures, images, fonts, videos, sounds, and so on).
The index.html is kind of like the "home page" of your WebGL experience. But, in most cases, this will be the only "page" (unless you're trying to make multiple WebGL scenes that users can move between - mostly only relevant if you're aiming to create a full WebGL website, for example).
In the context of WebGL, index.html will consist of code that manufactures the full experience:
-
What's the size of the canvas the experience will be displayed in?
-
What's the color of this canvas? Is there a 360 "skybox" photo instead?
-
What type of camera displays the experience? Is it interactive? Does it animate on its own? Where is it placed in the 3D world?
-
What type of lights light-up the scene? How strong? What color?
-
What 3D models am I using in the experience? Where are they placed? Do they animate? Are they interactive? What kind of shaders & materials & textures thay have?
-
What sound files am I using? What triggers them? What volume?
-
Etc, etc.
We'll experiment with actual coding later-on in this lecture.
But, if you understand the concept, you might have figured out that in order to fire up your WebGL experience, you need to link the index.html file to your WIX HTML iframe box.
This is simpler than you think.
Storing On The Cloud
For the sake of this lecture we're going to use Amazon AWS.
This cloud service has a million features, but let's focus on its storage tool - Amazon S3.
-
Sign-up to Amazon AWS (you'll need a credit card).
-
Amazon AWS has a free-tier program for 12 months, so you're not gonna be paying much of anything (for now).
-
After signing in to your console, search for S3 in the search bar and enter it:

4. In the new window, click on the blue button - Create Bucket.
5. Now give it a name, and select the region you believe most of your users come from.
Click Next. Then Next again.
6. Under "Set Permissions", it is important to disable "block all public access", since we want users to be able to see our stored WebGL experiences, via our WIX website:
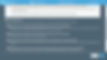
7. Create the bucket and enter it.
8. Click the "Create Folder" blue button and give it a unique name.
9. Click the "Upload" blue button, and drag all files relevant to your WebGL experience - the index.html, the 3D models, the textures, the sounds, etc, etc.
Once you get more into coding, the arrangement of these files into sub-folders could be important. We'll skip that for now.
10. Under "Set Permissions", and under "Manage public permissions", change to "Grant public read access to this object(s)":
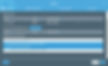
11. Click Next, Next, and Upload. Let it finish uploading to 100% with no errors.
12. Now click on your uploaded index.html file, it will look like this:

13. Copy the Object URL.
14. Back in your WIX Editor, enter the copied url into your HTML iframe:
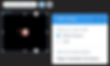
15. Tadaa! You now have your WebGL experience running in your WIX website!
Wait, You Said There's
A Way To Make Interactive WebGL Without Coding?
Yes, with a concept called Visual Scripting.
Visual scripting means that instead of actually writing your code, you use pre-defined "boxes" that each represents a different coding function. If you connect them together in a certain logic, you will get a working code - as if you wrote it yourself:

Thing is, those "boxes" don't just connect on their own - you need to do that for them. And so, visual scripting still requires that you understand the principles & concepts of the coding language.
For beginners, this makes coding easier, more intuitive & faster. But if you're serious about coding - you shouldn't go down this path as you will find it quite limiting!
An interesting software that uses visual scripting for WebGL is called Verge3D.
Let's go over it for a sec:
VERGE3D
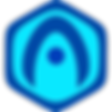
Verge3D has a unique set-up - it works like a plugin to an existing 3D software like Blender or Maya, and allows you to tranform 3D scenes that you build in, into full WebGL experiences.
Since you're already going to learn a 3D software (right??), this is a great solution.
To make the scenes interactive, Verge3D uses their own version of visual scripting, called "Puzzles". It's kind of like those code boxes, but with puzzle pieces:
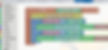
Verge3D also provides you with a few code examples, to get you inspired:
Here's a few neat examples of interactive WebGLs created using Verge3D:
Using Game Engines
To Create WebGL Experiences


Professional game engines, like Unity or Unreal, provide you with top-of-the-line tools to create truly stunning & complex games and experiences for all platforms - PC, Mac, Mobile, Xbox, Playstation, VR & AR Headsets, and yes, even for WebGL.
With some fantastic optimization tools, you can create very rich experiences that will still be able to run on your browser.
But, and it's a big BUT, these game engines are not built on Javascript. To code in Unity you're going to be using C#, and to code in Unreal you're going to be using C++.
When you export to WebGL, your project (automatically) translates into Javascript.
This method, and the fact the game engines are so extensive & complex, leaves a large "foot-print" on the size of your WebGL.
Exporting an empty WebGL scene from Unity creates a 5MB project, for example.
That's a lot of megabytes for a cube!
This is the reason you should NEVER create simple WebGL experiences (like 3D Viewers or Interactive Backgrounds) using game engines, because of the longer loading times.
ONLY use them if you're aiming to make a very complex WebGL, where 5MB doesn't make much of a difference.
BTW, both game engines support visual scripting, but learning to code in them will give you a much better understanding of how they work and what is possible.
They both also have a huge store with free & paid plugins, that can extend your capabilities by a mile.
If you're debating which game engine to choose for WebGL, Unreal has made that decision for you, as they recently deprecated their support of "simple WebGL exporting".
That means you can't export your scene as WebGL, upload the scene's files & index.html to the cloud, and link it to your WIX website (as I explained before).
You can only display WebGL experiences from Unreal by using a feature called Pixel Streaming. With this tool, you "stream" your game into the website, from the cloud.
There's no loading times, and there's no reliance on the users' computers' hardware at all.
While this is very cool, this type of streaming is VERY EXPENSIVE, as you pay for every hour the experience runs live on your website.
You should only use this if you have the backing of a large funding, or if you're creating a "timed" website, like a virtual event that only lasts a few days.
With Unity, you can also use a paid service to have cloud streaming (www.furioos.com), but Unity also supports the "simple WebGL exporting" method (which is free, with no time limit).
Here's an example of a work-in-process Virtual Art Exhibition that was created using Unity.
Notice it holds a real 3D laser scanned building, with 8K textures and full user interactivity.
The aim is to gamify the online art showcasing experience and immerse you inside.
-
Look around with your Mouse.
-
Walk around using the keypads - W S A D.
-
Jump with the Spacebar.
-
Crouch with keypad C.
-
Pickup objects and interact with your Left Mouse Button.
-
Examine objects using your Right Mouse Button.
-
Open a Menu with keypad ESC, and click to teleport to different parts.
-
Etc, etc.
And if we're on the subject of 3D scans & web, here's a recent development by the New York Times,
which shows their plans to use 3D scanning of real places, in order to enhance journalistic storytelling: